God class (also called Monster Class) is a class that single-handedly implements large blocks of functionality within a system and performs multiple, non-cohesive functionalities. It violates the basic idea of structured programming which is: Break a big problem into many smaller problems, solve those, and the combination of results obtained inevitably solves the big problem. A God class contains much overall functionality in a single object, holds too much data and has too many methods. God classes are referred to as being the object-oriented analogy of failing to use subroutines in procedural programming languages.
Impact
- A God class tends to become bulky and complex.
- Refactoring becomes a fairly complicated task. Refactoring of such a class affects a significant part of the system, as other members of the system naturally depend heavily on God classes.
- The tendency to hold too much non-cohesive functionality also means this class becomes critical; making even a small change to it has a system-wide impact in terms of testing efforts.
- Time and maintenance cost increases.
Characteristics
- The class is large and complex (high LOC and too many long and complex methods).
- Methods implement non-cohesive functionalities.
public class BankAccount { private AccountInfo acInfo; // Cohesive methods public void openAccount(); public void closeAccount(); // Non-cohesive method (should ideally exist in Customer class) public void createCustomer(); ...
//Class BankAccount accesses attributes of AccountInfo class // directly private AccountInfo acInfo; // Class also implements logic that should belong to AccountInfo class. public boolean isAccountValid() { if( (acInfo.isActive) && (acInfo.balance > acInfo.minimum balance ) { return true; } return false; }
public boolean isAccountValid() { return acInfo.isAccountValid(); }
Example(s)
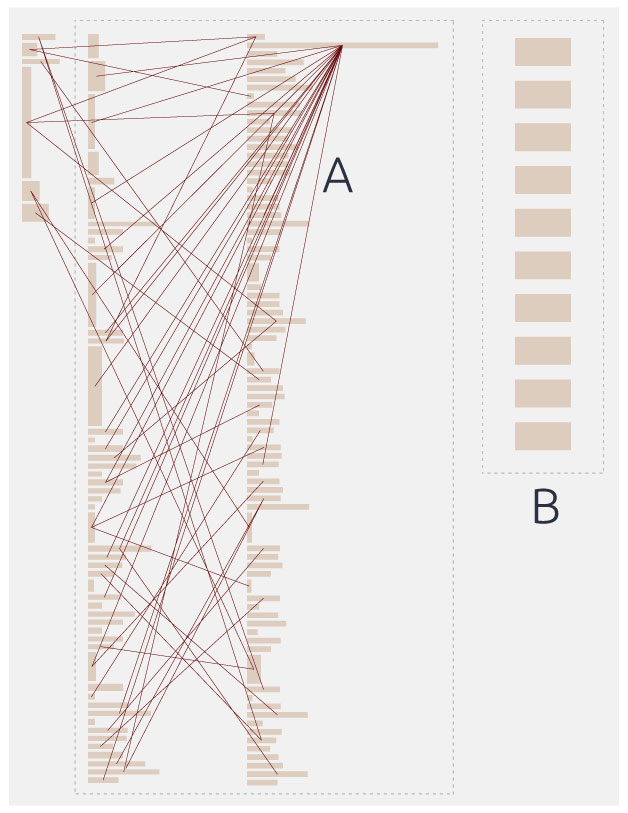
Statistics of Jedit
- The class has 143 methods and 31 attributes.
- The class accesses (directly or by getter/setter methods) about 28 attributes from 13 different classes – a characteristic of God Class.
- Methods seem to be long and complex.
- Methods have many branches (Total of 447 branches).
Inferred from the diagram above
- Public Interface and Internal Implementation layers:
- The class has too many methods (143). Methods seem to be complex.
- Attributes layer:
- Too many attributes (33)
- None of the class methods are accessing any of the attributes.
- This is a symptom of lack of sharing of common class level parameters.
Guidelines
- Follow the ‘one class one task’ rule. Distribute the system intelligence across several classes and let one class handle only one task. Collectively they will solve the intended problem.
- Functionalities that have common, overlapping aspects but are also dissimilar in other aspects can be implemented as sibling classes derived from a common base class.
- If distinct groups of data-operations exist in one class, try extracting them into separate classes.
- Make sure that class methods are not too long and do not use more than necessary data from other classes.
- God class is often a result of incrementally adding functionality to an existing class over time. This should be avoided. Try refactoring the class occasionally. Split the class instead of adding new functionality.